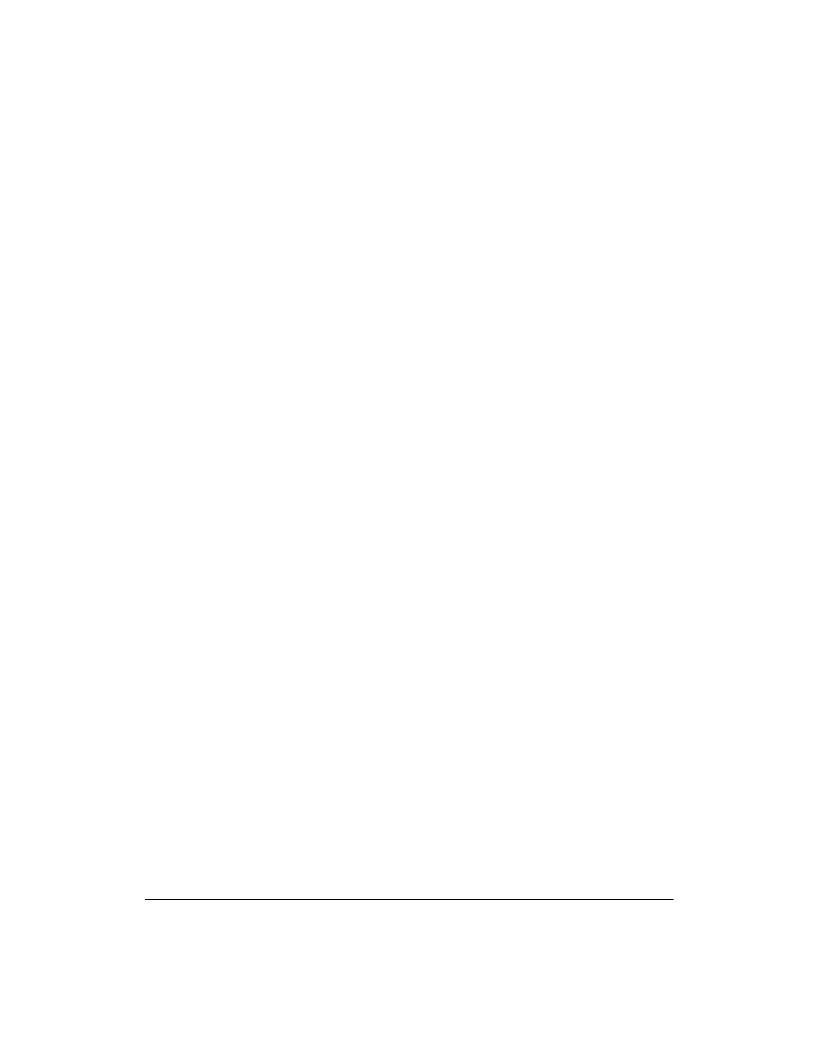
93
Chapter 2 Applications Programming
Although the activation record can be any size within the limits of the physical
cache, the compiler will not allocate more than 16 registers to the parameter-passing
part of the activation record. Functions that cannot pass all of their outgoing parame-
ters in registers must use a memory stack for additional parameters; global register
msp
(
gr125
) points to the top of the memory stack. This happens infrequently, but is
required for parameters that have their address taken (for example in C,
&variable
).
Data parameters at known addresses cannot be supported in register address space
because data addresses always refer to memory, not to registers.
The following code shows part of the
printf()
function for the 29K family:
printf:
sub
asgeu
add
. . .
jmpi
asleu
gr1,gr1,16
V_SPILL,gr1,rab ;compare with top of window
lr1,gr1,36
;rab is gr126
;function prologue
lr0
V_FILL,lr1,rfb
;return
;compare with bottom
;of window gr127
The register stack pointer,
rsp,
points to the bottom of the current activation re-
cord. All local registers are referenced relative to
rsp
. Four new registers are required
to support the function call shown, so
rsp
is decremented 16 bytes. Register
rsp
per-
forms a role similar to the MC68000’s
A7
and
A6
registers, except that it points to data
in high-speed registers, not data in external memory.
The compiler reserves local registers
lr0
and
lr1
for special duties within each
activation record. The
lr0
contains the execution starting address when it returns to
the caller’s activation record. The
lr1
points to the top of the caller’s activation re-
cord, the new frame allocates local registers
lr2
and
lr3
to hold
printf()
function local
variables.
As Figure 2-2 shows, the positions of five registers overlap. The three
printf()
parameters enter from
lr2
,
lr3
and
lr4
of the caller’s activation record and appear as
lr6
,
lr7
and
lr8
of the
printf()
function activation record.
2.1.3 Spilling And Filling
If not enough registers are available in the cache when it moves down the regis-
ter stack, then a V_SPILL trap is taken, and the registers spill out of the cache into
memory. Only procedure calls that require more registers than currently are available
in the cache suffer this overhead.
Once a spill occurs, a fill (V_FILL trap) can be expected at a later time. The fill
does not happen when the function call causing the spill returns, but rather when
some earlier function that requires data held in a previous activation record (just be-
low the cache window) returns. Just before a function returns, the
lr1
register, which
points to the top of the caller’s activation record, is compared with the pointer to the